mybatis 官网:https://mybatis.org/mybatis-3/zh/index.html
简介
MyBatis 是一款优秀的持久层框架,它支持自定义 SQL、存储过程以及高级映射。MyBatis 免除了几乎所有的 JDBC 代码以及设置参数和获取结果集的工作。MyBatis 可以通过简单的 XML 或注解来配置和映射原始类型、接口和 Java POJO(Plain Old Java Objects,普通老式 Java 对象)为数据库中的记录。
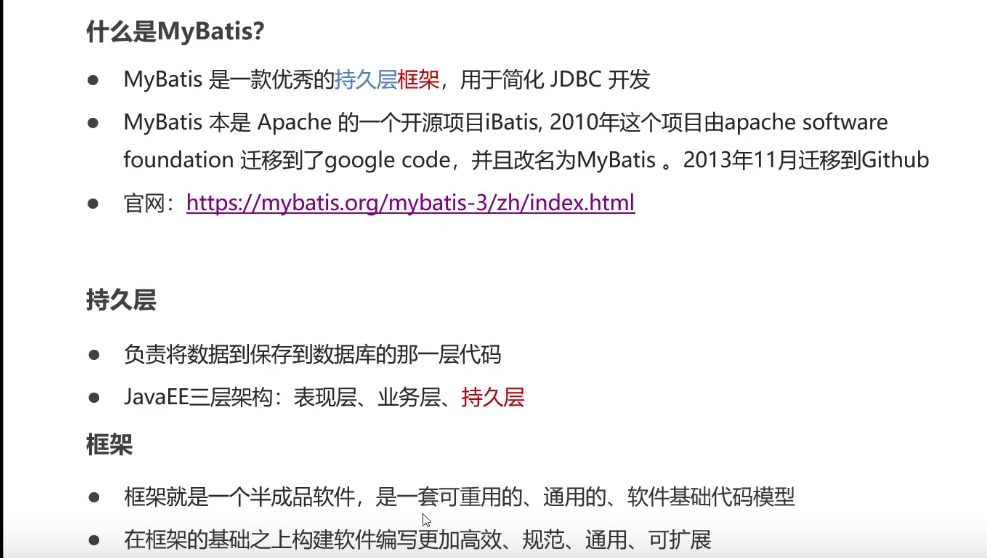
JDBC缺点
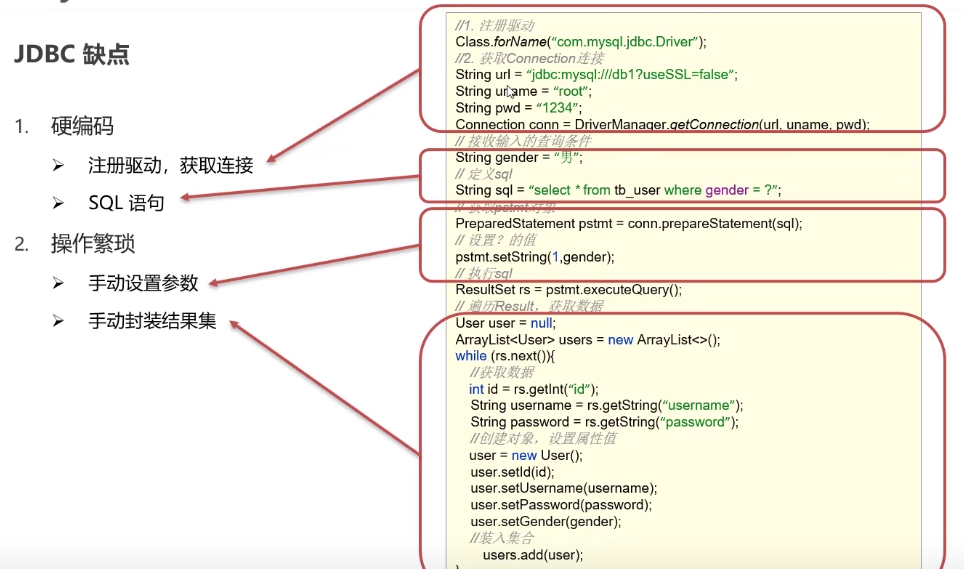
MyBatis 简化
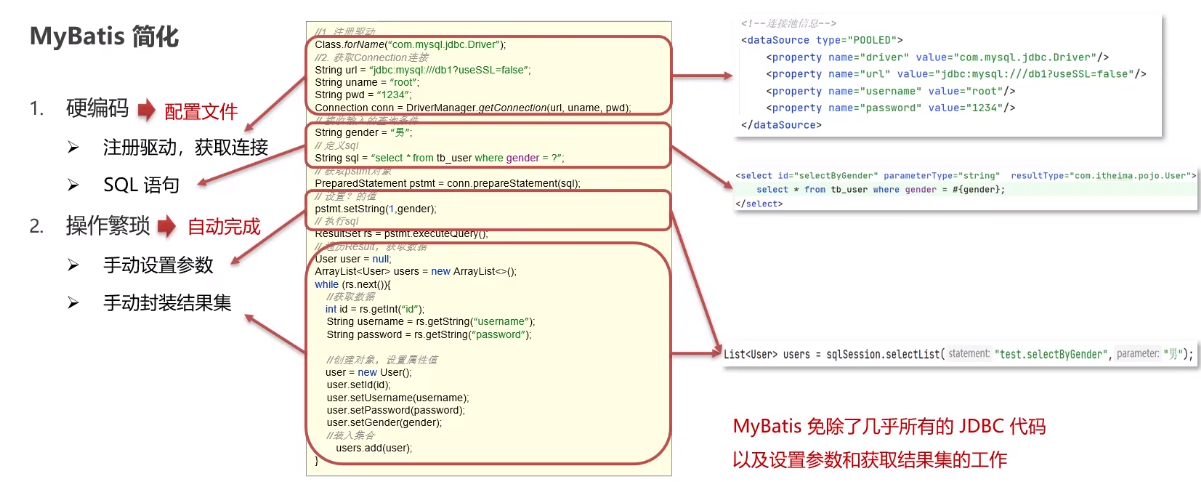
MyBatis 快速入门
package com.quange; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.io.InputStream; import java.util.List; /** * Mybatis 快速入门 */ public class MyBatisDemo { public static void main(String[] args) throws IOException { // 加载mybatis 核心配置文件,获取对象 String resource = "mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); // 获取sqlSession对象 SqlSession sqlSession = sqlSessionFactory.openSession(); // 执行sql语句 List<Object> users = sqlSession.selectList("test.selectAll"); System.out.println(users); // 释放资源 sqlSession.close(); } }
mybatis-config.xml 核心配置文件
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd"> <configuration> <environments default="development"> <environment id="development"> <transactionManager type="JDBC"/> <dataSource type="POOLED"> <!--数据库连接信息--> <property name="driver" value="com.mysql.cj.jdbc.Driver"/> <property name="url" value="jdbc:mysql:///quange?useSSL=false"/> <property name="username" value="root"/> <property name="password" value="123456"/> </dataSource> </environment> </environments> <mappers> <!--加载sql映射文件--> <mapper resource="userMapper.xml"/> </mappers> </configuration>
<?xml version="1.0" encoding="UTF-8" ?> <!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> <!-- namespace:名称空间 --> <mapper namespace="test"> <!--查询user表中所有数据,并返回User对象--> <select id="selectAll" resultType="com.quange.pojo.User"> select * from user; </select> </mapper>
Mapper 代理开发
解决原生方式中的硬编码
简化后期执行sql
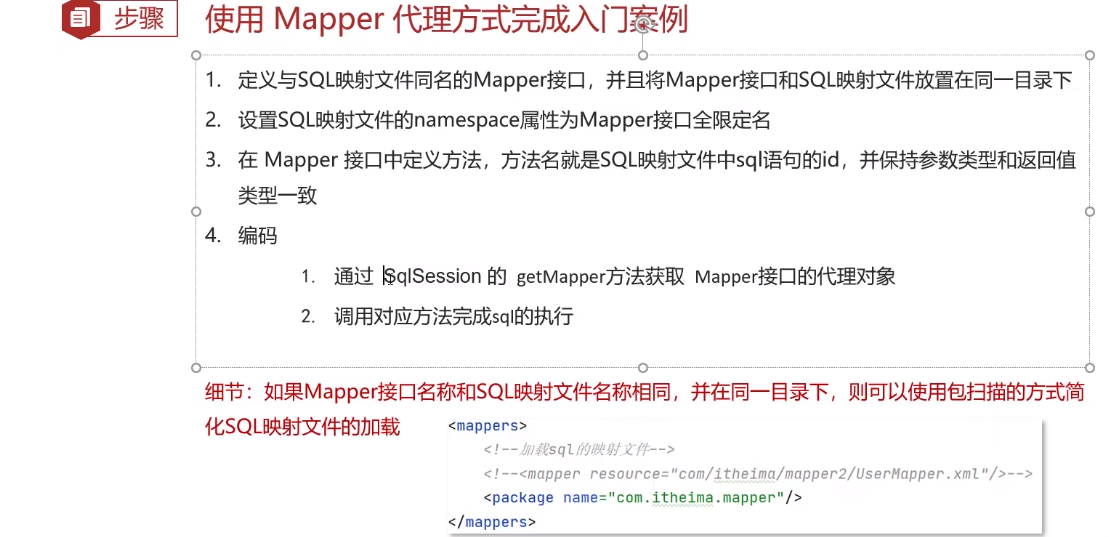
package com.quange; import com.quange.mapper.UserMapper; import com.quange.pojo.User; import org.apache.ibatis.io.Resources; import org.apache.ibatis.session.SqlSession; import org.apache.ibatis.session.SqlSessionFactory; import org.apache.ibatis.session.SqlSessionFactoryBuilder; import java.io.IOException; import java.io.InputStream; import java.util.List; /** * Mybatis 代理开发 快速入门 */ public class MyBatisDemo2 { public static void main(String[] args) throws IOException { // 加载mybatis 核心配置文件,获取对象 String resource = "mybatis-config.xml"; InputStream inputStream = Resources.getResourceAsStream(resource); SqlSessionFactory sqlSessionFactory = new SqlSessionFactoryBuilder().build(inputStream); // 获取sqlSession对象 SqlSession sqlSession = sqlSessionFactory.openSession(); // 执行sql语句,获取对应的UserMapper接口的代理对象 UserMapper userMapper = sqlSession.getMapper(UserMapper.class); List<User> users = userMapper.selectAll(); System.out.println(users); // 释放资源 sqlSession.close(); } }
mybatis 核心配置文件
官网:https://mybatis.org/mybatis-3/zh/configuration.html
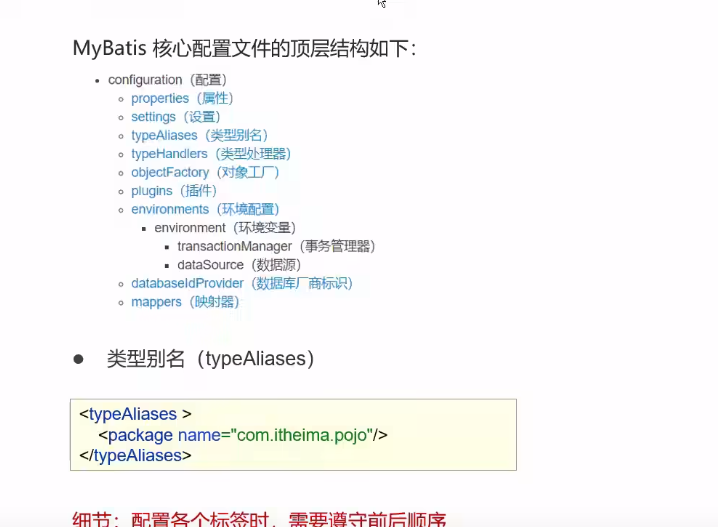
配置文件完成增删改查
查
MyBatisX 插件
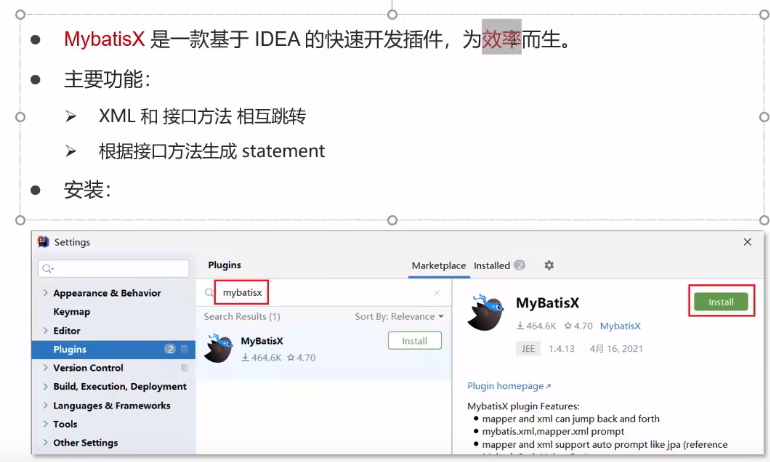
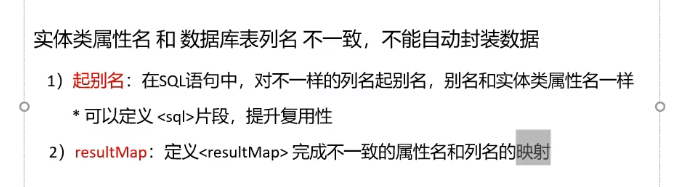
<resultMap id="brandResultMap" type="com.quange.pojo.Brand"> <!-- id: 完成主键字段的映射 column:表的列名 property: 实体类的属性名 result: 完成一般字段的映射 column:表的列名 property: 实体类的属性名 --> <result column="brand_name" property="brandName" /> <result column="company_name" property="companyName" /> </resultMap> <select id="selectAll" resultMap="brandResultMap"> select * from tb_brand; </select>
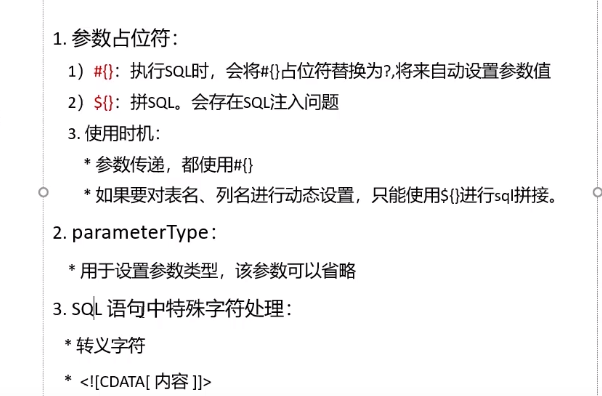
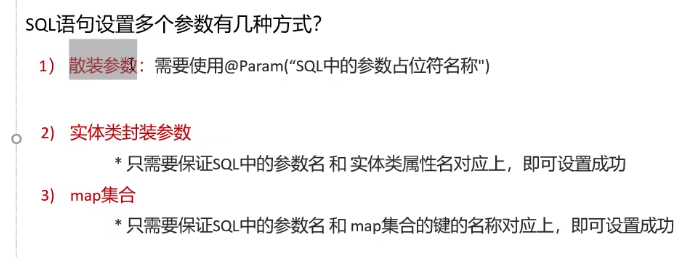
动态条件查询
动态 SQL 是 MyBatis 的强大特性之一。如果你使用过 JDBC 或其它类似的框架,你应该能理解根据不同条件拼接 SQL 语句有多痛苦,例如拼接时要确保不能忘记添加必要的空格,还要注意去掉列表最后一个列名的逗号。利用动态 SQL,可以彻底摆脱这种痛苦。
使用动态 SQL 并非一件易事,但借助可用于任何 SQL 映射语句中的强大的动态 SQL 语言,MyBatis 显著地提升了这一特性的易用性。
如果你之前用过 JSTL 或任何基于类 XML 语言的文本处理器,你对动态 SQL 元素可能会感觉似曾相识。在 MyBatis 之前的版本中,需要花时间了解大量的元素。借助功能强大的基于 OGNL 的表达式,MyBatis 3 替换了之前的大部分元素,大大精简了元素种类,现在要学习的元素种类比原来的一半还要少。
- if
- choose (when, otherwise)
- trim (where, set)
- foreach
<!-- 动态条件查询 --> <select id="selectByCondition" resultMap="brandResultMap"> select * from tb_brand <where> <if test="status != null"> status = #{status} </if> <if test="companyName != null and companyName!= ''"> and company_name like #{companyName} </if> <if test="brandName != null and brandName != ''"> and brand_name like #{brandName}; </if> </where> </select>
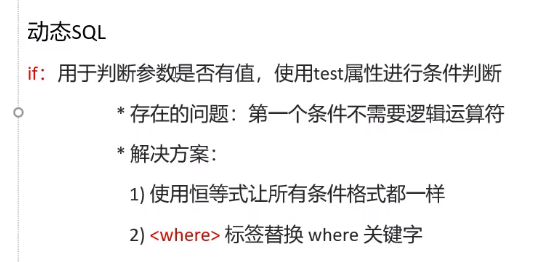
单条件,动态条件查询

<!--单条件 动态查询 --> <select id="selectByConditionSingle" resultMap="brandResultMap"> select * from tb_brand <where> <choose> <when test="status != null"> status = #{status} </when> <when test="companyName != null and companyName!= ''"> company_name like #{companyName} </when> <when test="brandName != null and brandName != ''"> brand_name like #{brandName}; </when> </choose> </where> </select>
添加

主键返回
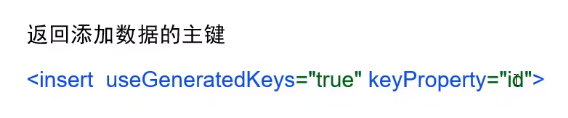
<!--添加数据--> <insert id="addSingle" useGeneratedKeys="true" keyProperty="id"> insert into tb_brand (brand_name, company_name, ordered, description, status) values (#{brandName}, #{companyName}, #{ordered}, #{description}, #{status}); </insert>
修改
修改全部字段
<!-- 修改全部数据--> <update id="update"> update tb_brand set brand_name = #{brandName}, company_name = #{companyName}, ordered = #{ordered}, description = #{description}, status = #{status} where id = #{id} </update>
修改动态字段
使用 set 标签和if标签,实现动态修改
<!-- 动态修改数据 --> <update id="updateDynamic"> update tb_brand <set> <if test="brandName != null and brandName != ''"> brand_name = #{brandName}, </if> <if test="companyName != null and compandName != ''"> company_name = #{companyName}, </if> <if test="ordered != null"> ordered = #{ordered}, </if> <if test="description != null and description != '' "> description = #{description}, </if> <if test="status != null"> status = #{status}, </if> </set> where id = #{id}; </update>
删除
删除单个数据
<!-- 删除单个数据 --> <delete id="delById"> delete from tb_brand where id = #{id}; </delete>
删除多个数据
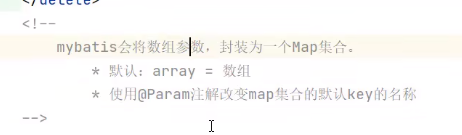
<!-- 删除多个数据--> <delete id="delByIds"> delete from tb_brand where id in <foreach collection="ids" item="id" separator="," open="(" close=")"> #{id} </foreach> ; </delete>
版权声明:
一、本站致力于为软件爱好者提供国内外软件开发技术和软件共享,着力为用户提供优资资源。
二、本站提供的所有下载文件均为网络共享资源,请于下载后的24小时内删除。如需体验更多乐趣,还请支持正版。
三、我站提供用户下载的所有内容均转自互联网。如有内容侵犯您的版权或其他利益的,请编辑邮件并加以说明发送到站长邮箱。站长会进行审查之后,情况属实的会在三个工作日内为您删除。
如若转载,请注明出处:https://www.quange.cc/note/java/java-web/1466.html
共有 0 条评论