Set接口
Set接口基本介绍
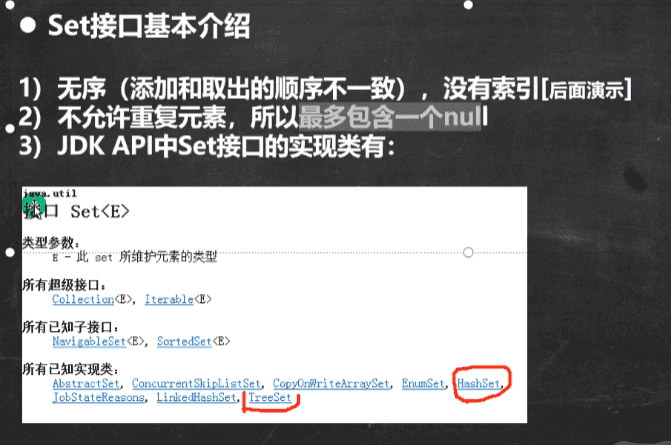
Set接口的常用方法

Set接口的遍历方式
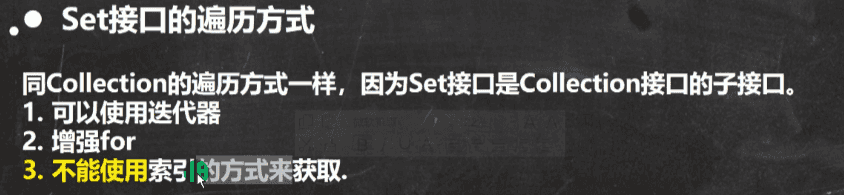
HashSet类的全面说明
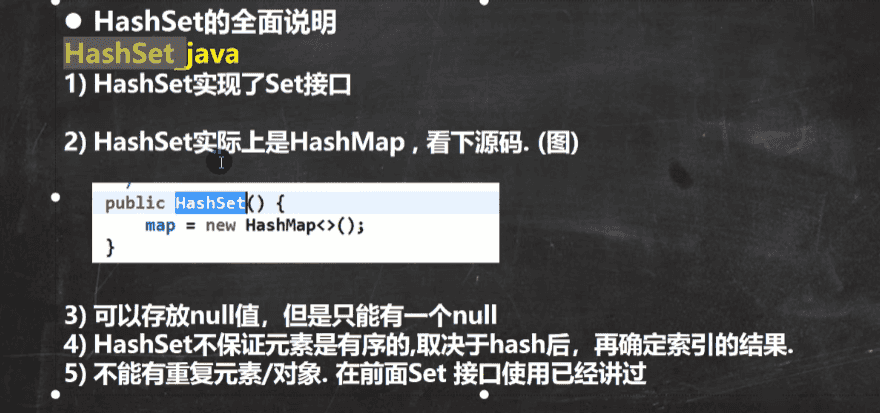
HashSet 底层机制说明

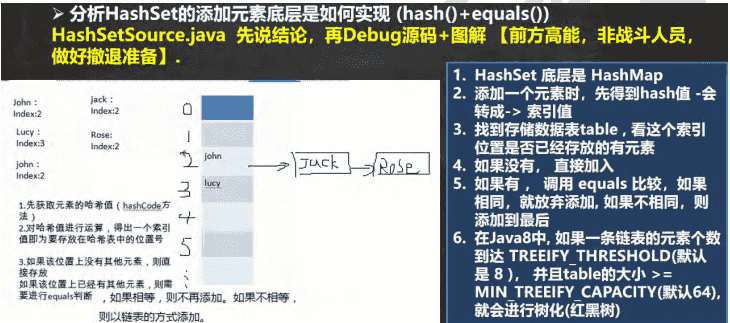
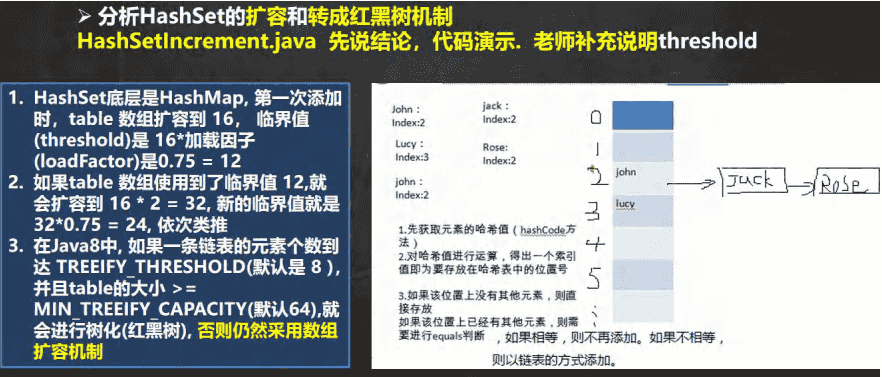
课后练习题
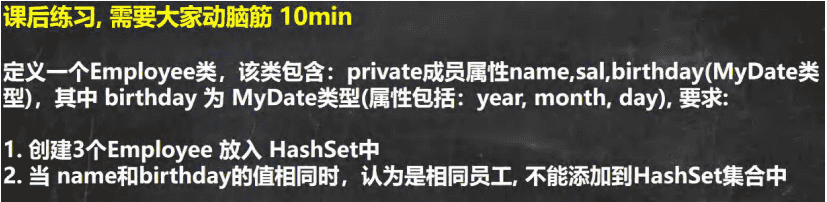
实现代码
package com.quange.test; import java.util.HashSet; import java.util.Objects; public class test06 { public static void main(String[] args) { HashSet hashSet = new HashSet(); hashSet.add(new Employee("tom", 2000, new MyDate(2022,1,2))); hashSet.add(new Employee("sal", 3000, new MyDate(2022,1,3))); hashSet.add(new Employee("tom", 2000, new MyDate(2022,1,2))); System.out.println(hashSet); } } class Employee{ private String name; private int sal ; private MyDate birthday; public Employee(String name, int sal, MyDate birthday) { this.name = name; this.sal = sal; this.birthday = birthday; } public String getName() { return name; } public void setName(String name) { this.name = name; } public int getSal() { return sal; } public void setSal(int sal) { this.sal = sal; } public MyDate getBirthday() { return birthday; } public void setBirthday(MyDate birthday) { this.birthday = birthday; } // 重写equals 方法,判断name 和 birthday @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; Employee employee = (Employee) o; return Objects.equals(name, employee.name) && Objects.equals(birthday, employee.birthday); } // 重写hashCode 方法,判断name 和birthday @Override public int hashCode() { return Objects.hash(name, birthday); } // 重写toString方法 @Override public String toString() { return "Employee{" + "name='" + name + '\'' + ", sal=" + sal + ", birthday=" + birthday + '}'; } } class MyDate{ private int year; private int month; private int day; public MyDate(int year, int month, int day) { this.year = year; this.month = month; this.day = day; } public int getYear() { return year; } public void setYear(int year) { this.year = year; } public int getMonth() { return month; } public void setMonth(int month) { this.month = month; } public int getDay() { return day; } public void setDay(int day) { this.day = day; } // 重写 equals 方法,判断年月日 @Override public boolean equals(Object o) { if (this == o) return true; if (o == null || getClass() != o.getClass()) return false; MyDate myDate = (MyDate) o; return year == myDate.year && month == myDate.month && day == myDate.day; } // 重写 hashCode 方法,判断年月日 @Override public int hashCode() { return Objects.hash(year, month, day); } // 重写 toString 方法 @Override public String toString() { return "MyDate{" + "year=" + year + ", month=" + month + ", day=" + day + '}'; } }
输出,第三个对象未能添加
[Employee{name='tom', sal=2000, birthday=MyDate{year=2022, month=1, day=2}}, Employee{name='sal', sal=3000, birthday=MyDate{year=2022, month=1, day=3}}]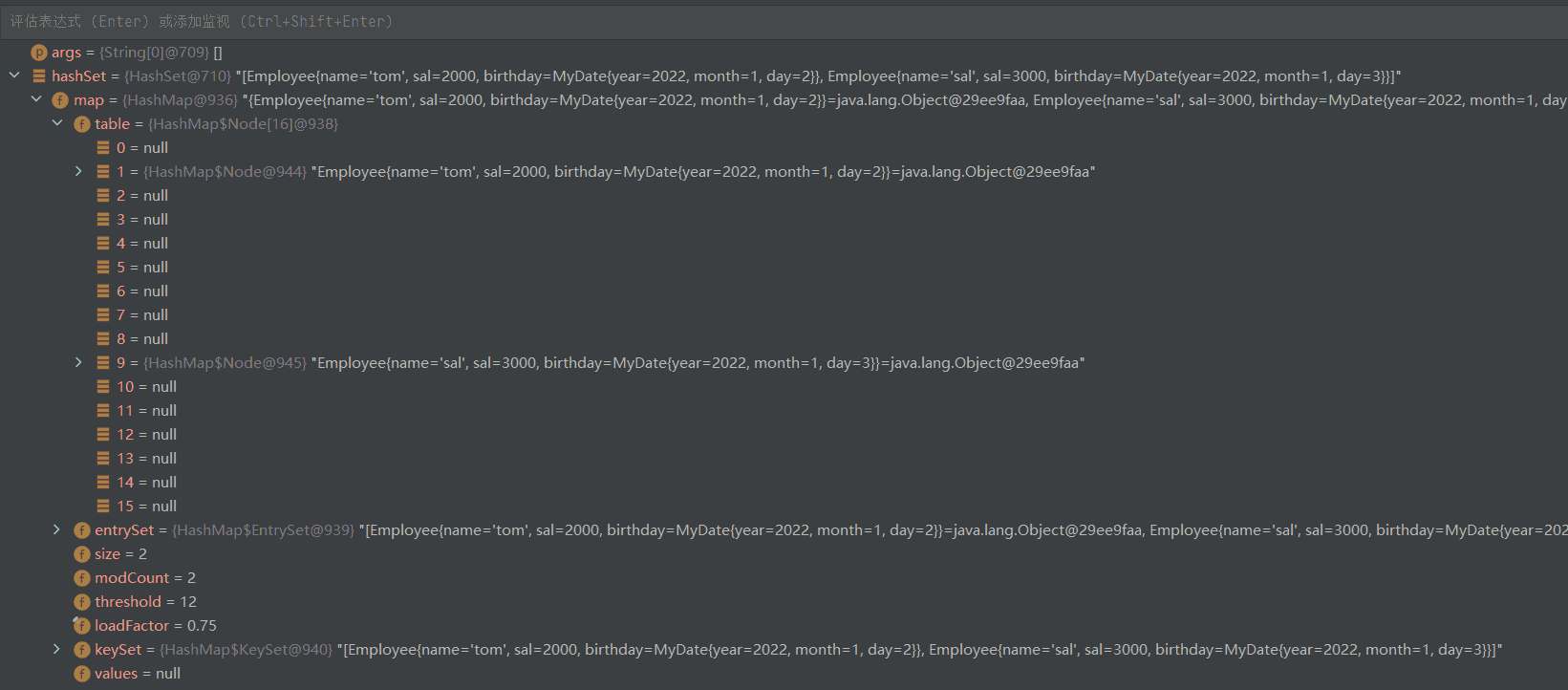
- THE END -
最后修改:2022年4月24日
版权声明:
一、本站致力于为软件爱好者提供国内外软件开发技术和软件共享,着力为用户提供优资资源。
二、本站提供的所有下载文件均为网络共享资源,请于下载后的24小时内删除。如需体验更多乐趣,还请支持正版。
三、我站提供用户下载的所有内容均转自互联网。如有内容侵犯您的版权或其他利益的,请编辑邮件并加以说明发送到站长邮箱。站长会进行审查之后,情况属实的会在三个工作日内为您删除。
如若转载,请注明出处:https://www.quange.cc/note/java/learn-java/1076.html
共有 0 条评论